All Class
12 Programs.
Explore
Code
07 November 2023 Stack
1. WAP to show stacks in python.
s=[] # a stack is basically a list open from one side only - aka the end
# This is a menu driven program, yours doesnt need to
def push(x):
s.append(x)
return "done!"
while True:
print("""
+----------------------+
| |
| SELECT ACTION |
| |
+----------------------+
| |
| [1] Push |
| [2] Pop |
| [3] Display |
| [4] Exit |
| |
+----------------------+
ↆ Enter your choice """)
c=int(input(""))
if c==1:
inp=int(input("Enter element >> "))
out=push(inp)
print(inp)
if c==2:
out=s.pop() #yes, popping a stack is the same as a list
print("Element removed:", str(out))
if c==3:
print(s[::-1]) # bcz stacks open from the ending
if c==4:
break
This website is now archived, new comments can't be posted.
24 July 2023 File Handling // Day 9 - CSV files.
1. WAP to Create a csv file of student details.
import csv
f = open("data.csv", "w",newline="") # without the newline argument - it creates a new line when we enter a new record
wr = csv.writer(f)
n=int(input("Enter number of records you wish to add -> "))
wr.writerow(["R. NO.", "NAME", "CLASS", "SECTION"])
for i in range(n):
r=int(input("Enter roll number -> "))
n=input("Enter name -> ")
c=int(input("Enter class -> "))
s=input("Enter section -> ")
wr.writerow([r,n,c,s])
f.close()
This website is now archived, new comments can't be posted.
2. WAP to add a record in a csv file of student details.
import csv
f = open("data.csv", "r") # without the newline argument - it creates a new line when we enter a new record
c = csv.reader(f)
l=[]
for i in c:
l.append(i)
f.close()
n=int(input("Enter number of records you wish to add -> "))
f = open("data.csv", "w",newline="")
wr = csv.writer(f)
for i in range(n):
r=int(input("Enter roll number -> "))
n=input("Enter name -> ")
c=int(input("Enter class -> "))
s=input("Enter section -> ")
l.append([r,n,c,s])
for i in l:
wr.writerow(i)
f.close()
This website is now archived, new comments can't be posted.
3. WAP to remove a record in a csv file of student details.
import csv
f = open("data.csv", "r") # without the newline argument - it creates a new line when we enter a new record
c = csv.reader(f)
l=[]
for i in c:
l.append(i)
for i in l:
for j in i:
print(j,end="\t")
print()
f.close()
n=input("Enter roll number of the student who's record you wish to remove -> ")
f = open("data.csv", "w",newline="")
wr = csv.writer(f)
for i in l:
if i[0]!=n:
wr.writerow(i)
f.close()
This website is now archived, new comments can't be posted.
4. WAP to edit a record in a csv file of student details.
import csv
f = open("data.csv", "r") # without the newline argument - it creates a new line when we enter a new record
c = csv.reader(f)
l=[]
for i in c:
l.append(i)
for i in l:
for j in i:
print(j,end="\t")
print()
f.close()
n=input("Enter roll number of the student who's record you wish to edit -> ")
s=0
for i in l:
if i[0]==n:
s=1
print(" Enter field to modify\n 1 - Name\n 2 - Class\n 3 - Section")
mode=int(input(" -> "))
if mode==1:
h=input(" Enter new name ->")
i[1]=h
elif mode==2:
h=int(input(" Enter new class ->"))
i[2]=h
elif mode==3:
h=input(" Enter new section ->")
i[3]=h
else:
print(" invalid input!")
break
else:
s=0
if s==1:
f = open("data.csv", "w",newline="")
wr = csv.writer(f)
for i in l:
wr.writerow(i)
f.close()
This website is now archived, new comments can't be posted.
5. WAP to search a record in a csv file of student details.
import csv
f = open("data.csv", "r")
c = csv.reader(f)
l=[]
for i in c:
l.append(i)
f.close()
n=input("Enter the roll number of student who's record you wish to search -> ")
x=0
for i in l:
if i[0]==n:
x=1
for j in i:
print(j,end="\t")
print()
else:
x=0
if x==0:
print("Record not found!")
This website is now archived, new comments can't be posted.
6. WAP to list all records in a csv file of student details.
import csv
f = open("data.csv", "r") # without the newline argument - it creates a new line when we enter a new record
c = csv.reader(f)
for i in c:
for j in i:
print(j,end="\t")
print()
f.close()
This website is now archived, new comments can't be posted.
21 July 2023 File Handling // Day 8 - Practice Questions.
1. WAP to count the number of Uppercase letters in a text file.
Lorem ipsum dolor sit amet, Consectetur adipiscing elit.
Nam tempus, arcu in dapibus Iaculis, felis sapien congue Felis, et pretium lectus.
f=open("text2.txt","r")
s=f.read()
c=0
for i in s:
if i.isupper():
c+=1
print("Number of Uppercase letters ->",c)
f.close()
This website is now archived, new comments can't be posted.
2. WAP to count the number of Uppercase letters in a text file per line.
Lorem ipsum dolor sit amet, Consectetur adipiscing elit.
Nam tempus, arcu in dapibus Iaculis, felis sapien congue Felis, et pretium lectus.
f=open("text2.txt","r")
l=f.readlines()
for i in range(len(l)):
c=0
for j in l[i]:
if j.isupper():
c+=1
print("Number of Uppercase letters in line", i+1, "->",c)
f.close()
This website is now archived, new comments can't be posted.
19 July 2023 File Handling // Day 7 - Binary files.
1. WAP to insert a record into a binary file. (Without deleting the old data, no you can't use append - it won't work.)
No file preview available.
import pickle as p
f=open("shinto.dat","rb")
l=[]
while True:
try:
h=p.load(f)
l.append(h)
except EOFError:
f.close()
break
n=input("Enter name ->")
c=int(input("Enter class ->"))
r=int(input("Enter roll no ->"))
s=input("Enter stream ->")
l.append([r,n,c,s])
f=open("shinto.dat","wb")
for j in l:
p.dump(j,f)
f.close()
This website is now archived, new comments can't be posted.
Anmol Sharma
actually, my bad, append does work and you could use it. I think back when we tried doing it in class it did not work, so we conclude that appending in binary is broken.
18 July 2023 File Handling // Day 6 - Binary files.
1. A file result.dat has names with their marks in english, maths and CS. Write a function called display() to display all students who scored 90+ in all 3 subjects.
result.dat [processed]
[['Anmol',93,94,95],['Raghav',95,96,97],['Daksh',87,83,92]]
import pickle as p
def result():
f=open("result.dat","rb")
l=[]
while True:
try:
rec=p.load(f)
l.append(rec)
except:
f.close()
break
for i in l:
if i[1]>=90 and i[2]>=90 and i[3]>=90:
for j in i:
print(j,end="\t")
print()
result()
This website is now archived, new comments can't be posted.
Arya
aree how rude
Anmol Sharma
heehee
14 July 2023 File Handling // Day 5 - Binary files.
1. WAP to delete the records of the binary file created the previous day.
No file preview available.
import pickle as p
f=open("shinto.dat","rb")
l=[]
print("R. NO.\tNAME\tCLASS\tSTREAM")
while True:
try:
rec=p.load(f)
l.append(rec)
r,n,c,s=rec
print(r,n,c,s,sep="\t")
except:
f.close()
break
f=open("shinto.dat","wb")
rno=int(input("Enter roll number of student to be removed -> "))
for j in l:
if j[0]==rno:
pass
else:
p.dump(j,f)
f.close()
This website is now archived, new comments can't be posted.
Akshay Tushir
gore csv ke codes kha hai
Anmol Sharma
bhai 😭 i was busy.. daal dunga
13 July 2023 File Handling // Day 4 - Binary files.
1. WAP to search the records of the binary file created the previous day.
No file preview available.
import pickle as p
f = open("shinto.dat", "rb")
l = []
n = int(input("Enter roll number -> "))
# implement searching based on other factors
while True:
try:
rec = p.load(f)
l.append(rec)
except:
f.close()
break
print("R. NO.\tNAME\tCLASS\tSTREAM")
for i in l:
if i[0] == n:
r, n, c, s = i
print(r, n, c, s, sep="\t")
This website is now archived, new comments can't be posted.
2. WAP to modify the records of the binary file created the previous day.
No file preview available.
# the one im showing here is quite a complicated version, you can go for something simple
import pickle as p
f=open("shinto.dat","rb")
l=[]
while True:
try:
rec=p.load(f)
l.append(rec)
r,n,c,s=rec
print(r,n,c,s,sep="\t")
except:
f.close()
break
n=int(input("Enter Roll number of student whos data is to be modified -> "))
s=0
for i in l:
if i[0]==n:
s=1
print(" Enter field to modify\n 1 - Name\n 2 - Class\n 3 - Stream")
mode=int(input(" -> "))
if mode==1:
h=input(" Enter new name ->")
i[1]=h
elif mode==2:
h=int(input(" Enter new class ->"))
i[2]=h
elif mode==3:
h=input(" Enter new stream ->")
i[3]=h
else:
print(" invalid input!")
break
else:
s=0
if s==1:
f=open("shinto.dat","wb")
for i in l:
p.dump(i,f)
f.close()
else:
print("Record not found!")
This website is now archived, new comments can't be posted.
12 July 2023 File Handling // Day 3 - Binary files.
1. WAP to take lists of data and add them to a binary file
by Aryaman Sharma
No file preview available.
import pickle
f=open("shinto.dat","wb")
l=[]
while True:
n=input("Enter name ->")
if n.lower()=="end":
break
c=int(input("Enter class ->"))
r=int(input("Enter roll no ->"))
s=input("Enter stream ->")
l=[r,n,c,s]
pickle.dump(l,f)
f.close()
This website is now archived, new comments can't be posted.
2. WAP to read the binary file just created.
No file preview available.
import pickle as p
f=open("shinto.dat","rb")
print("R. NO.\tNAME\tCLASS\tSTREAM")
while True:
try:
r,n,c,s=p.load(f)
print(r,n,c,s,sep="\t")
except:
f.close()
break
This website is now archived, new comments can't be posted.
Arya
\n hona chahiye for tabular format in line 6
Anmol Sharma
woo sep="\t" hai, matlab har varieable kee biich mein tab space hogi. end character \n hii hai chutiye
4 July 2023 Revision // Practice Questions.
1. WAP to take a string from the user till the user enters 'END'. Save the data to a file "myfile.txt" and display only sentences starting with Uppercase alphabets.
question by Aryaman Sharma
f=open("myfile.txt","w")
l=""
while True:
inp=input("-> ")
if inp!="END":
l=l+inp+"\n" #we use `\n` to create a new line
else:
f.write(l)
f.close()
break
g=open("myfile.txt","r")
s=g.readlines()
for i in s:
if i[0].isupper():
print(i,end="")
g.close()
This website is now archived, new comments can't be posted.
Akshay Tushir
Good one joe
Anmol Sharma
racist motherfucker
2. Write a function to take a string as a parameter and return the number of vowels as a dictionary.
question by Aryaman Sharma
def count(a):
d={"a":0,"e":0,"i":0,"o":0,"u":0}
for i in a:
if i in "aeiouAEIOU":
c=d.get(i)
d.update({i.lower():c+1})
return d
print(count("random string"))
This website is now archived, new comments can't be posted.
3 July 2023 File Handling // Day 2 - Practice Questions.
1. WAP to count the number of words in a text file.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam tempus, arcu in dapibus iaculis, felis sapien congue felis, et pretium lectus.
f=open("textfile.txt","r")
s=f.read()
l=s.split()
print("Number of words ->",len(l))
f.close()
This website is now archived, new comments can't be posted.
2. WAP to count the number of words starting with a particular ("a" for this example) letter in a text file.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam tempus, arcu in dapibus iaculis, felis sapien congue felis, et pretium lectus.
f=open("textfile.txt","r")
s=f.read()
l=s.split()
c=0
for i in l:
if i[0]=="a":
c+=1
print("Number of words starting with 'a' ->",c)
f.close()
This website is now archived, new comments can't be posted.
3. WAP to create a copy of a text file.
original.txt
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam tempus, arcu in dapibus iaculis, felis sapien congue felis, et pretium lectus.
copy.txt
f=open("original.txt","r")
s=f.read()
g=open("copy.txt","w")
g.write(s)
g.close()
f.close()
This website is now archived, new comments can't be posted.
10 May 2023 File Handling // Day 1 - The basics.
1. WAP to create a text file.
f=open("file.txt","w")
f.write("abc")
f.close()
This website is now archived, new comments can't be posted.
2. WAP to change all characters of a file to UPPERCASE.
Just a test file
y'know
f=open("abc.txt","r")
s=f.read()
u=s.upper()
print(u)
This website is now archived, new comments can't be posted.
2. WAP to change the first letter of all words of a file to UPPERCASE.
Just a test file
y'know
f=open("abc.txt","r")
s=f.readlines()
for i in s:
for j in i.split():
print(j.capitalize(),end=" ")
print()
This website is now archived, new comments can't be posted.
8 May 2023 Functions // Day 7 - Question on math module.
1. WAP to find area of a regular polygon.
from math import tan,pi
s=int(input("Enter number of sides -> "))
l=int(input("Enter length of a side -> "))
ar=(s*l**2)/(4*tan(pi/s))
print(ar)
This website is now archived, new comments can't be posted.
2 May 2023 Functions // Day 6 - Functions + Dictionaries
1. WAP to create a dictionary.
def makedic(n):
l={}
for i in range(n):
h=eval(input("Enter key -> "))
k=eval(input("Enter value -> "))
l.update({h:k})
return l
print(makedic(2))
This website is now archived, new comments can't be posted.
28 April 2023 Functions // Day 5 - Global and Local scopes
1. Write a program to demonstrate local and global scopes.
by Aryaman Sharma
G=10 #glboal variable intially taken
def localvariable():
G=15 #this value is local therefore only in this function will the value of G be 15
return G
def joseph():
global G #global functions makes it so that G=20 is the new official value of G all over the code, calling is essential however
G=20
def biden():
global G
G=30
def borris():
global G
G=40
print(G) #yield should be 10 as origianl value of G was 10
joseph()
print(G) #yield should be 20
biden()
print(G) #yield should be 30
borris()
print(G) #yield should be 40
#although value of G is sustained for local for e.g.
print(localvariable())
This website is now archived, new comments can't be posted.
Anmol Sharma
welp, i forgot return from the localvariable function
Akshay Tushir
Nice name borris
Anmol Sharma
shutup akshay
Akshay Tushir
Bas itne hi programs hai na
Anmol Sharma
well, ek aur hai bas daal raha hun ruk jaa
26 April 2023 Functions // Day 4 - Returning multiple values from a function
1. Create a function to add and subtract values and return the both of these values.
def func(a,b):
c=a+b
d=a-b
return c,d
a,b=func(420,69)
print(a,b,sep="\n")
This website is now archived, new comments can't be posted.
24 April 2023 Functions // Day 3 - Importing Functions
1. Import a function from a file.
def func(a,b):
c=a*b
return c
from modl import func
print(func(10,20))
This website is now archived, new comments can't be posted.
17 April 2023 Functions // Day 2 - User Defined Functions - Passing Args + Default arguments
1. Make some functions using args.
# function defs ->
def area_sqr(s=10):
ar=s*s
return ar
def area_rect(a=10,b=20):
ar=a*b
return ar
def addnum(a,b):
s=a+b
return s
def expo(a,b=2):
e=1
for i in range(b):
e*=a
return e
# function calls ->
print("Area of sqr:",area_sqr())
print("Area of sqr with args:",area_sqr(200))
print("Area of rect:",area_rect())
print("Area of rect with args:",area_rect(60,90))
print("Add num:",addnum(7,2))
print("Exponent:",expo(3))
print("Exponent with second arg:",expo(3,5))
This website is now archived, new comments can't be posted.
13 April 2023 Functions // Day 1 - User Defined Functions
1. Make 3 functions: area of square, area of rectangle and sum of 2 numbers
def area_sqr():
s=10
print("Area of square:", s*s)
def area_rect():
a=10
b=20
print("Area of rectangle:", a*b)
def add():
a=69
b=420
print("Add:", a+b)
area_sqr()
area_rect()
add()
This website is now archived, new comments can't be posted.
5 April 2023 Review of Python // Day 4 - Lists, Touples and Dictionaries
1. WAP to take input from user in list.
l=[]
n=int(input("Enter no. -> "))
for i in range(n):
l.append(int(input("-> ")))
print(l)
This website is now archived, new comments can't be posted.
2. WAP to take input from user in touple.
t=()
n=int(input("Enter no. -> "))
for i in range(n):
t+=(int(input("-> ")),)
print(t)
This website is now archived, new comments can't be posted.
3. WAP to find min-max in list.
l=[]
n=int(input("Enter no. -> "))
for i in range(n):
l.append(int(input("-> ")))
print(l)
maxm=0
for i in l:
if i>maxm:
maxm=i
minm=maxm
for j in l:
if j < minm:
minm=j
print("Maximum ->", maxm)
print("Minimum ->", minm)
This website is now archived, new comments can't be posted.
4. WAP to take input from user in dictionary.
d={}
n=int(input("Enter no. of entries -> "))
for i in range(n):
na=input("Enter name -> ")
cl=int(input("Enter class -> "))
rn=int(input("Enter roll no. -> "))
mh=int(input("Maths -> "))
ph=int(input("Physics -> "))
ch=int(input("Chemistry -> "))
l=[cl,rn]
t=(mh,ph,ch)
d.update({na:[l,t]})
print(d)
This website is now archived, new comments can't be posted.
5. Sort a list
l=[6,9,4,2,0]
for i in range(len(l)):
for j in range(i-1,-1,-1):
if l[j+1] < l[j]:
l[j],l[j+1]=l[j+1],l[j]
print(l)
This website is now archived, new comments can't be posted.
3 April 2023 Review of Python // Day 3 - Strings
1. String functions
#a test string
# Bro = Mr. sharma btw
t="Bros IQ is room temperature in celsius scale"
# .isalpha(): returns true if the tring has only alphabets
print("abc".isalpha())
print("abc ".isalpha())
print("abc21".isalpha())
# .isdigit(): True if string has only digits
print("123".isdigit())
# len(): length of string (spaces are also counted)
print(len("hi :D"))
# .split(seperator, maxsplit): string to list
print(t.split())
print(t.split("e"))
print(t.split("e", 3)) #['Bros IQ is room t', 'mp', 'ratur', ' in celsius scale'] -> notice how it does not split on the e in celsius
# .lower(): Converts all upper case letters to lower case
print(t.lower())
# .islower: Checks if every char is in lower case
print("abc 123 sbs".islower())
# .upper(): Converts all lower case letters to upper case
print(t.upper())
# .isupper: Checks if every char is in upper case
print("ABC 123 SDS".isupper())
# .find(substring, start, end): true if the given substing is found in the given range
print(t.find("i"))
print(t.find("i", 9))
print(t.find("i", 0, 2)) # -1 if not found
# .lstrip(string): remove the given substr from the left of the string or " " if no argument is given
print(" ab".lstrip())
print("hhhhhhxy".lstrip("h"))
# .rstrip(string): remove the given substr from the right of the string or " " if no argument is given
print("ab ".rstrip())
print("xy hhhhhhhhhhh".rstrip("h"))
print(" ab".lstrip())
print("xy ".lstrip("c")) #returns the same substr?
# .isspace(): BRO, THIS SHIT LITERALLY CHECKS IF THE STRING IS A SPACE. LIKE YOU COULD DO S==" "
print(" ".isspace())
print(" obviously not".isspace())
# .istitle(): checks if a str is a title. Oh what is a title? well the first letter of wach word must be upper case and no special characters.
print("This".istitle())
print("This is not".istitle())
print("This is @lso not".istitle())
print("But This Is".istitle())
# "substr".join(str): tbh very confusing, it takes in a string and joins each char in it with the given substr
# substr will be what u want to seperate each char with
# and str will be the main string
print("#".join(t)) # you might be reminded of something from here
# .swapcase(): It swaps upper case chars for lower case ones and vise-versa
print(t.swapcase())
# .partition(str): splits the string into 3 parts and returns a touple
print(t.partition("IQ")) #notice how it returns a touple with 3 portions
# 69TH LINE GG
# .endswith(substr): checks if the string ends with the given substr
print("abc 123 xyz".endswith("xyz"))
print("abc 123 xyz".endswith("abc"))
# .startswith(substr): checks if the string starts with the given substr
print("abc 123 xyz".startswith("xyz"))
print("abc 123 xyz".startswith("abc"))
# ord(): ordinal value for string
print(ord("A"))
# chr(): character value for ordinal
print(chr(69))
#thats it
This website is now archived, new comments can't be posted.
2. Take a string from the user and replace space with # (according to someone, you really GOTTA TAKE THE # FROM THE USER TOO, YEAHHHH)
s=input("Enter string -> ")
l=s.replace(" ","#")
print(l)
This website is now archived, new comments can't be posted.
20 March 2023 Review of Python // Day 2
1. WAP to calculate the income tax of employee on the basis of basic sallery and total savings subject to these conditions:
(i) If sallery is less than 2.5 L, no tax
(ii) If sallery is b/w 2.5 L and 5 L, 5% tax
(iii) If sallery is b/w 5 L and 10 L, 20% tax
(iv) If sallery is greater than 10 L, 30% tax
(v) On an investment of upto 1.5 L, employee cam save 45 K
b=int(input("Enter basic pay -> "))
s=int(input("Enter saving -> "))
t=0
if b<=250000:
t=0
elif 250000<=b<=500000:
t=0.05*b
elif 500000<=b<=1000000:
t=0.2*b
else:
t=0.3*b
if t>=150000:
if t>s:
t=t-45000
else:
if t>s:
t=t-s
print(t)
This website is now archived, new comments can't be posted.
14 March 2023 Review of Python // Day 1
1. Some guy (forgot his name) borrowed Rs. 40,000 from some other guy. He wants to repay it after 6 years. Find the total amount he has to pay using simple interest. (bro literally gave us a math question in CS 💀💀)
p=40000 #principal amnt
r=8 #rate of interest
t=6 #time in yrs
print("Total amount at the end of 6 years:",p+((p*r*t)//100))
This website is now archived, new comments can't be posted.
2. Generate fibonacci series using while loop.
n=int(input("Enter the number of terms: "))
a=0
b=1
i=1
while i<=n:
c=a+b
print(c)
a=b
b=c
i+=1
This website is now archived, new comments can't be posted.
3. Find a raised to the power b.
a=int(input("Enter number -> "))
b=int(input("Enter power -> "))
ans=1
for i in range(1,b+1):
ans*=a
print(a,"^",b,"=",ans)
This website is now archived, new comments can't be posted.
Anmol Sharma
test
4. Print prime numbers between 2 & 200.
for i in range(2,201):
prm=True
for j in range(2,(i//2)+1):
if i%j!=0:
prm=True
else:
prm=False
break
if prm==True:
print(i)
else:
continue
This website is now archived, new comments can't be posted.
Anmol Sharma
h
Anmol Sharma
hi
colguy
hi :3
Anmol
Oh damn
Before you proceed...
As insane as this might sound, we haven't really done anything for SQL or SQL connectivity in the class. We did do the theory part but that's as far as it goes, so I'm posting a few SQL programs from my side similar to the ones we discussed in class.
If you're wondering why you can't run the code in browser for this tab, I haven't worked on that feature, and I have no plans to, and you shouldn't expect me to.
How the content on this page works
Here's what I'm doing: I'll be creating the database you see below and then use it to perform some queries. I assume you have just launched MySQL and haven't loaded into any databases.
I also expect you to know about the different MySQL commands.
+---------+--------------+-------+---------+-------+
| roll_no | name | class | section | marks |
+---------+--------------+-------+---------+-------+
| 101 | John Doe | 10 | A | 85 |
| 102 | Jane Smith | 11 | B | 92 |
| 103 | Mike Johnson | 9 | C | 78 |
| 104 | Sarah Brown | 12 | A | 95 |
| 105 | Alex Davis | 10 | B | 89 |
| 106 | Emily White | 11 | C | 88 |
| 107 | David Lee | 10 | A | 91 |
| 108 | Jessica Chen | 9 | B | 76 |
+---------+--------------+-------+---------+-------+
(Dummy data provided by ChatGPT)
1. Creating and using a database
01 >> Creating a database
create database studentdb;
02 >> Using the database just created
use studentdb;
This website is now archived, new comments can't be posted.
2. Creating a table with a primary key.
create table student(
roll_no int not null primary key,
name varchar(30) not null,
class int,
section varchar(10),
marks int
);
You can view the structure of the table by running
DESCRIBE student;
This website is now archived, new comments can't be posted.
3. Inserting data into a database
01 >> Method 1 - Without changing positions
INSERT INTO student VALUES (101, 'John Doe', 10, 'A', 85);
02 >> Method 2 - By changing positions
INSERT INTO student (roll_no, name, marks, class, section) VALUES (102, 'Jane Smith', 92, 11, 'B');
03 >> Inserting multiple records
INSERT INTO student VALUES
(103, 'Mike Johnson', 9, 'C', 78),
(104, 'Sarah Brown', 12, 'A', 95),
(105, 'Alex Davis', 10, 'B', 89),
(106, 'Emily White', 11, 'C', 88),
(107, 'David Lee', 10, 'A', 91),
(108, 'Jessica Chen', 9, 'B', 76);
This website is now archived, new comments can't be posted.
4. Viewing all records
SELECT * FROM STUDENT;
This website is now archived, new comments can't be posted.
Now that the table is created, we'll perform some queries on it.
5. View only the Roll number and name columns
SELECT ROLL_NO, NAME FROM STUDENT;
This website is now archived, new comments can't be posted.
6. Filtering records using specific conditions and where clauses
01 >> Select all columns for records having marks less than 90
SELECT * FROM STUDENT WHERE MARKS<90;
02 >> Select all columns for records having roll numbers 103 to 107
SELECT * FROM STUDENT WHERE ROLL_NO BETWEEN 103 AND 107;
03 >> Select all columns for records having names starting with J
SELECT * FROM STUDENT WHERE NAME LIKE "J%";
04 >> Select all columns for records having names starting with J and having 7 characters precisely
SELECT * FROM STUDENT WHERE NAME LIKE "J______";
05 >> Select Marks for all students and subtract 10 from it
SELECT NAME, MARKS - 10 FROM STUDENT;
This website is now archived, new comments can't be posted.
7. Order By
01 >> Select all records and arrange them alphabetically by name.
SELECT * FROM STUDENT ORDER BY NAME;
02 >> Select all records and arrange them in dicreasing order of marks
SELECT * FROM STUDENT ORDER BY MARKS DESC;
This website is now archived, new comments can't be posted.
8. Group By
01 >> Display the number of Students in each class. Also order the result by class.
SELECT CLASS, COUNT(*) FROM STUDENT GROUP BY CLASS ORDER BY CLASS;
This is a very simple example, I suggest you to explore something more detailed from our textbook.
This website is now archived, new comments can't be posted.
Here we covered the different queries which are usually used. Now, I'm gonna discuss updating records and Altering the structure of the table.
9. Updating records
01 >> Update the record of Emily White and set their section to B.
update student
set section="B"
where roll_no=106;
02 >> Update everyone's record and reduce their marks by 10
update student
set marks=marks-10;
This website is now archived, new comments can't be posted.
10. Altering table
01 >> Insert a new column "fee" with a default value
alter table student
add fee int default 3000;
02 >> Rename the column "fee" to "t_fee"
alter table student
rename column fee to t_fee;
03 >> Change the data type of t_fee to varchar
alter table student
modify column t_fee varchar(10);
04 >> Delete the t_fee column
alter table student
drop column t_fee;
This website is now archived, new comments can't be posted.
And finally, we got deleting a record and dropping a table.
01 >> Delete the record of anyone whose name is Alex Davis
DELETE FROM STUDENT WHERE NAME="Alex Davis";
02 >> Drop the table
DROP TABLE STUDENT;
This website is now archived, new comments can't be posted.
Oh hey! looks like someone read this all through, now that you're here consider reading the textbook and solving some questions from there.
Before you proceed...
As insane as this might sound, we haven't really done anything for SQL or SQL connectivity in the class. We did do the theory part but that's as far as it goes, so I'm posting a few SQL connectivity programs from my side similar to the ones we discussed in class.
If you're wondering why you can't run the code in browser for this tab, I haven't worked on that feature, and I have no plans to, and you shouldn't expect me to.
How the content on this page works
For this page, I'll be using the table I created on the SQL page (visit that before reading this). I'm gonna write some programs performing varying queries on the below table. I will also try to cover as many concepts as possible in my code.
Database name: studentdb // Table name: student
+---------+--------------+-------+---------+-------+
| roll_no | name | class | section | marks |
+---------+--------------+-------+---------+-------+
| 101 | John Doe | 10 | A | 85 |
| 102 | Jane Smith | 11 | B | 92 |
| 103 | Mike Johnson | 9 | C | 78 |
| 104 | Sarah Brown | 12 | A | 95 |
| 105 | Alex Davis | 10 | B | 89 |
| 106 | Emily White | 11 | C | 88 |
| 107 | David Lee | 10 | A | 91 |
| 108 | Jessica Chen | 9 | B | 76 |
+---------+--------------+-------+---------+-------+
(Dummy data provided by ChatGPT)
1. Show all records of the table.
import mysql.connector as m
con=m.connect(host="localhost", user="root", password="root", database="studentdb")
cur=con.cursor()
# executing select cmd
cur.execute("SELECT * FROM STUDENT")
res=cur.fetchall()
print("Roll No","Student Name","Class","Section","Marks",sep="\t")
for i in res:
for j in i:
print(j,end="\t")
print()
This website is now archived, new comments can't be posted.
2. Insert a new record into the table.
import mysql.connector as m
con=m.connect(host="localhost",user="root", password="root", database="studentdb")
cur=con.cursor()
while True:
r=int(input("Enter Roll number -> "))
n=input("Enter name -> ")
c=int(input("Enter class -> "))
s=input("Enter section -> ")
m=int(input("Enter narks -> "))
data=(r,n,c,s,m)
cur.execute("INSERT INTO STUDENT VALUES(%s,'%s',%s,'%s',%s)"%data)
con.commit()
inp=input("Enter more records (yes/no)? ")
if inp.lower()=="yes":
continue
elif inp.lower()=="no":
break
else:
print("Inavlid input - exitting input menu")
break
This website is now archived, new comments can't be posted.
3. Search all records based on specific parameters.
import mysql.connector as m
con=m.connect(host="localhost",user="root",password="root",database="studentdb")
cur=con.cursor()
print("""What field do you want the search to proceed with?
1 > Roll Number
2 > Name
3 > Class
4 > Section
5 > Marks""")
pm=int(input("\n>>> "))
if pm==1:
q=(int(input("Enter Roll number -> ")),) #anything after % must be passed as a touple
cur.execute("SELECT * FROM STUDENT WHERE roll_no=%s"%q)
elif pm==2:
q=(input("Enter Name -> "),)
cur.execute("SELECT * FROM STUDENT WHERE name='%s'"%q)
elif pm==3:
q=(int(input("Enter Class -> ")),)
cur.execute("SELECT * FROM STUDENT WHERE class=%s"%q)
elif pm==4:
q=(input("Enter Section -> ").capitalize(),)
cur.execute("SELECT * FROM STUDENT WHERE section='%s'"%q)
elif pm==5:
q=(int(input("Enter Marks -> ")),)
cur.execute("SELECT * FROM STUDENT WHERE marks=%s"%q)
res=cur.fetchall()
print("Roll No","Student Name","Class","Section","Marks",sep="\t")
for i in res:
for j in i:
print(j,end="\t")
print()
This website is now archived, new comments can't be posted.
4. Modify a specific record (everything except roll number).
import mysql.connector as m
con=m.connect(host="localhost",user="root",password="root",database="studentdb")
cur=con.cursor()
r=int(input("Enter Roll number of the student who's record you want to edit -> "))
cur.execute("SELECT * FROM STUDENT WHERE roll_no={roll}".format(roll=r))
data=cur.fetchone()
print("\nRecord ---//\n")
print("Roll No","Student Name","Class","Section","Marks",sep="\t")
for i in data:
print(i,end="\t")
print("""\n\nWhich field do you want to edit?
1 > Name
2 > Class
3 > Section
4 > Marks""")
pm=int(input("\n>>> "))
if pm==1:
q=input("Enter new Name -> ")
cur.execute("UPDATE STUDENT SET name='{0}' WHERE roll_no={1}".format(q,r))
elif pm==2:
q=int(input("Enter Class -> "))
cur.execute("UPDATE STUDENT SET class={0} WHERE roll_no={1}".format(q,r))
elif pm==3:
q=input("Enter Section -> ").capitalize()
cur.execute("UPDATE STUDENT SET section='{0}' WHERE roll_no={1}".format(q,r))
elif pm==4:
q=int(input("Enter Marks -> "))
cur.execute("UPDATE STUDENT SET marks={0} WHERE roll_no={1}".format(q,r))
con.commit()
print("----[ RECORD UPDATED ]----")
This website is now archived, new comments can't be posted.
5. Delete a specific record.
import mysql.connector as m
con=m.connect(host="localhost",user="root",password="root",database="studentdb")
cur=con.cursor()
r=int(input("Enter roll number of record to delete -> "))
cur.execute("DELETE FROM STUDENT WHERE ROLL_NO={}".format(r))
con.commit()
print("----[ RECORD DELETED ]----")
This website is now archived, new comments can't be posted.
Yo hey! if you're here this probably means you've looked at all the code we did in class. Good job! *pat pat pat*
Copied to clipboard!
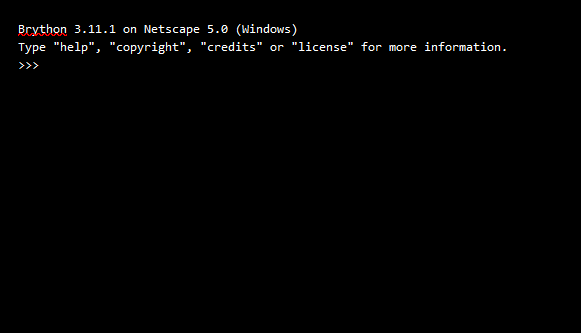
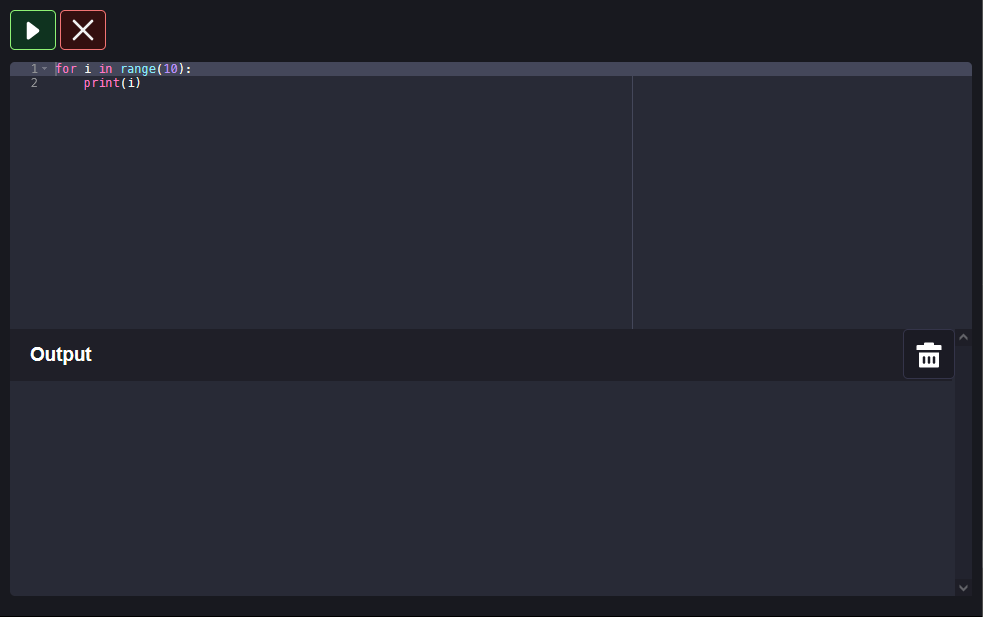
General Info
This website is open source (like 99% of my code) so people can insult me about shitty code. Here's the repo if you want: https://github.com/AnmolPlayzz/AC12P
Comment guidelines: https://github.com/AnmolPlayzz/AC12P/blob/main/COMMENT_GUIDELINES.md
Found a bug? Read this.
Have a suggestion? You can tell me on whatever the fuck app you want to use, but I'm probably too lazy to implement it. So yeah, feel free to waste your energy!
What did I use to make this website? As usual HTML (Hyper Text Markup Language), CSS (Cascading Style Sheets) and Javascript. Also mongodb to store the comments. btw SQL sucks I'm never gonna use it.
More often or not, people ask me why did I make this? Or rather some mfs say i made it for my "someone specil" even tho idk who tf this someone is, I just made it to store code for myself and to share it with the boiz. (Mr. Sharma and Mr. Arora, fuck off for this joke.)
Just a sidenote, I'm not an english nerd or something. Ignore my spelling mistakes. My brain ain't no grammerly or auto-correct. Basically, don't be grammar police.
Also, now that you're here, why not check out my website 💀. Yes this is an ad, shutup now.
Special thanks to Aryaman Sharma (iam.aryaman on insta) for giving me the programs on the days I was absent and for promoting this shit.
Licensed under The MIT License // https://github.com/AnmolPlayzz/AC12P/blob/main/LICENSE
Credits(awesome 3rd party libs that made this website possible)
Highlight.js: Highlighting code blocks/Syntax highlighting
Brython: Browser based executor for python
Ace: High performance code editor for the web
Netlify: Website hosting
Made with HTML, CSS, JavaScript, Python, Brython and 🥰 + 🎶 by @AnmolPlayzz (GitHub) // @anmolsharma_16 (Insta)
Version Info
LATEST | ARCHIVED
[10 March 2024] Version 4.11
What's new?
- I changed a few things on the home page and fixed a few bugs
This for sure is the final feature update on the website. It was super fun building it and maintaining. God, I wish time just went slower. Anyway this is a changelog, not the best place to dump my emotions.
[22 December 2023] Version 4.10
What's new?
- Added a tab UI to the code page to separate code from different languages.
This might as well be the final feature update on the website, assuming there are no bugs in my code.
edit: no
[05 August 2023] Version 4.9
What's new?
- An emulator for CSV files
[16 July 2023] Version 4.8
What's new?
- New home page (removed the 3D model bcz it was lagging the website on many devices)
- Raise the character limit for comments to precisely 969 characters (nice.)
[19 May 2023] Version 4.7
What's new?
- A file system emulator (i had to fucking make this thing and im so pissed rn, it just works for the file functions in python).
- Real time syncing for comments because ooo fancy tech.
- Fixed an indentation issue relating to brython (you should not give a fuck).
[9 May 2023] Version 4.6
What's new?
Comment guidelines (you must follow).
[1 May 2023] Version 4.5
What's new?
Comment feature (I want to explode)
[29 April 2023] Version 4.4
What's new?
New home page ballin' init?
[26 April 2023] Version 4.3.1
What's new?
A minor addition that emulates importing a python module (while running code in-browser) and will be later used for text files too cuz im lazy.
[14 April 2023] Version 4.3.0
What's new?
Migrated to 11ty framework (I'm dying inside).
[16 Mar 2023] Version 4.2.0
What's new?
Login and signup feature for a MASSIVE feature coming in future (requested by Mr. Sharma).
[3 Mar 2023] Version 4.1
What's new?
- UI update to web IDE
- Clear button for output. Thanks to breeze.me for the suggestion on discord.
[3 Mar 2023] Version 4.0.1
What's new?
- Auto hide drawer on clicking a link. Thnx to @shouko_komi for the suggestion on discord.
- Fixes for safari browser.
[2 Mar 2023] Version 4.0.0
What's new?
Major UI overhaul
[9 Feb 2023] Version 3.4.0
What's new?
A very early version of a python web IDE.
[8 Feb 2023] Version 3.3.2
What's new?
Broken code related to "edit in browser".
And wait for it, wait for it. I FUCKING SPELT MOTHERFUCKING PROGRAMS WRONG AS "PROGRAMMES" SINCE THE BEGINNING OF THIS SITE. Thnx to @__32 (wtf is this username) for pointing this out on Discord.
[8 Feb 2023] Version 3.3.1
What's new?
Minor fixes and remove that ugly piece of shit blue box you see when you click something on android/iOS.
[8 Feb 2023] Version 3.3.0
What's new?
Edit before Run feature
[5 Feb 2023] Version 3.2.1
What's new?
Fixed several bugs. Thnx to big man @blitzdrachen on discord for reporting 'em.
[5 Feb 2023] Version 3.2.0
What's new?
1. In browser Python terminal
2. Licensing info
[3 Feb 2023] Version 3.1.0
What's new?
Run in browser - run the programs in browser
[2 Feb 2023] Version 3.0.0
What's new?
New major release - UI update + Dark mode
Archival Notice
This website has been archived. Everything here is read only now.
Building this website has taught me a lot, about programming and about web design. So thank you to everyone who appreciated it! And even if you didn't like it, thanks for being a critic.
If you could take anything away from this, it's that dumb shit you make on a friday night can turn into something way cooler.
Special thanks to Aryaman Sharma (iam.aryaman on insta) for giving me the programs on the days I was absent and for promoting this shit.
- Anmol Sharma